Understanding the Fetch API
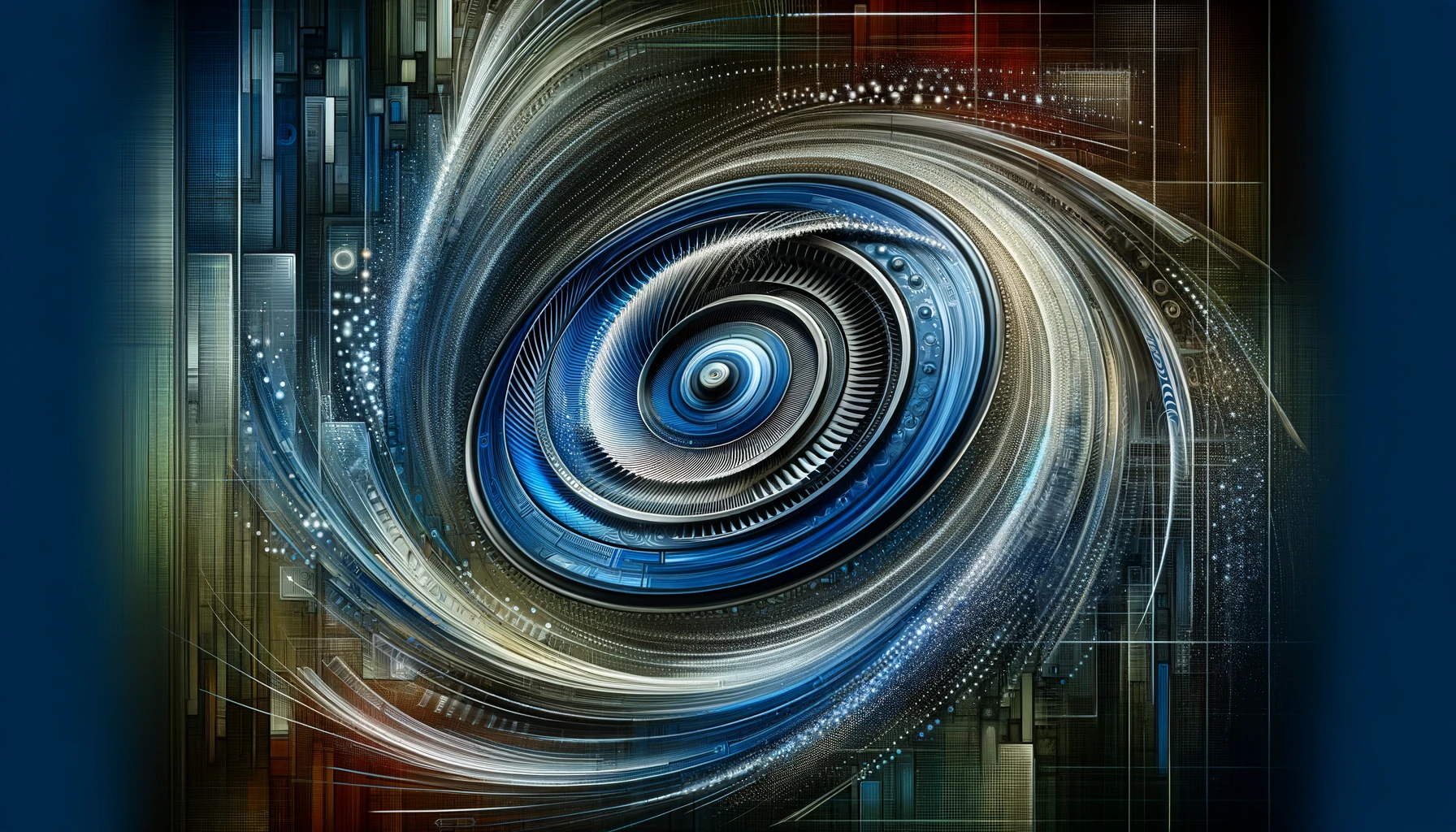
JavaScript's Fetch API provides a modern interface for making HTTP requests to servers, similar to the XMLHttpRequest but with a more versatile and powerful set of features. It is a crucial tool for web applications, allowing the user to request, send, and retrieve data. This article will discuss how Fetch works and explore key concepts such as synchronous vs. asynchronous operations, callback functions, and promises.
Understanding Synchronous vs. Asynchronous in JavaScript
In JavaScript, operations can be synchronous or asynchronous.
- Synchronous: Code is executed in sequence. Each statement waits for the previous one to finish before executing. This can lead to "blocking" on long-running operations.
- Asynchronous: Code can execute out of sequence, allowing long-running operations to complete without blocking the main thread. JavaScript often handles these with callbacks, promises, or async/await.
Callback Functions
A callback function is a type of function that is passed as an argument to another function. Callbacks are commonly used to handle the results of asynchronous operations. However, using callbacks can create complex nested code, sometimes called "callback hell."
Promises
Callbacks can be a hassle sometimes, but Promises offer a better solution. A Promise is an object that represents the completion or failure of an asynchronous operation. There are three possible states for a Promise:
- Pending: the initial state, meaning the operation hasn't been fulfilled or rejected yet.
- Fulfilled: the operation was successfully completed.
- Rejected: the operation failed.
Promises allow for better flow control and error handling with .then() for success and .catch() for errors.
Using the Fetch API
The Fetch API returns a Promise, making it easier to work with asynchronous requests.
Basic GET Request
Here's an example of a simple GET request using Fetch:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
POST Request with JSON
For a POST request, especially when sending JSON data, you need to set the method and headers appropriately:
fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
key1: 'value1',
key2: 'value2'
}),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Error Handling
Proper error handling with Fetch involves checking the response and throwing an error if the request isn't successful:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Async/Await
To make asynchronous code more readable, you can use async/await along with the Fetch API.
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData('https://api.example.com/data');
When combined with the power of promises and async/await, the Fetch API offers a modern and robust approach to making HTTP requests in JavaScript. It simplifies asynchronous programming, resulting in more manageable and readable code structures, especially when dealing with complex data retrieval or API integrations.